- A B=inherit(A);
- B B=A.inherit();
- C B.prototype=inherit(A);
- D B.prototype=inherit(A.prototype);
- Share this MCQ
In JavaScript, inheritance is implemented using prototypes, which are objects that serve as templates for creating other objects. There is no built-in inherit()
function in JavaScript that allows one class to inherit from another, but you can achieve inheritance by setting up the prototype chain correctly.
To inherit from a superclass in JavaScript, you can use the Object.create()
method to create a new object that has the superclass as its prototype. The new object will then inherit all of the properties and methods of the superclass.
For example:
function A() {
// Properties and methods of class A
}
A.prototype.sayHello = function() {
console.log('Hello from A');
}
function B() {
// Properties and methods of class B
}
// Set up inheritance
B.prototype = Object.create(A.prototype);
const b = new B();
b.sayHello(); // Output: "Hello from A"
Here, the B
class is defined as a constructor function. The B
prototype is then set to a new object that has the A
prototype as its prototype using the Object.create()
method.
This causes the B
prototype to inherit all of the properties and methods of the A
prototype, including the sayHello
method. When we create a new B
object using the new
keyword, the object inherits the sayHello
method from the B
prototype, which in turn inherited it from the A
prototype.
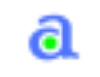
Share this MCQ