
Singly linked list in Data Structure
Table of Content:
Singly Linked List: C Programming Data Structure
- In this type of Linked List, two successive nodes are linked together in linear fashion.
- Each Node contains the address of the next node to be followed.
- In Singly Linked List only Linear or Forward Sequential movement is possible.
- Elements are accessed sequentially , no direct access is allowed.
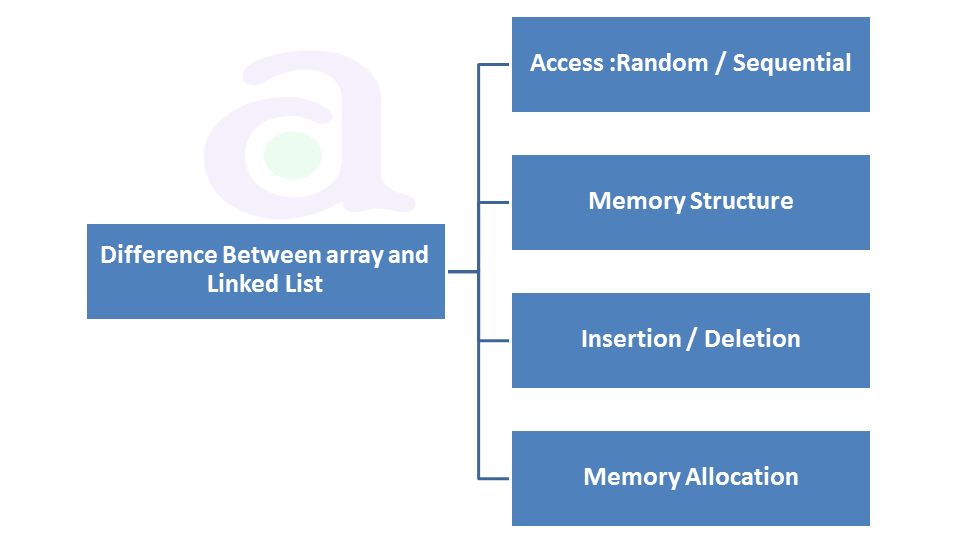
Explanation :
- It is the most basic type of Linked List in C.
- It is a simple sequence of Dynamically allocated Nodes.
- Each Node has its successor and predecessor.
- First Node does not have predecessor while the last node does not have any successor.
- Last Node has successor reference as "NULL".
- In the above Linked List We have 3 types of nodes.
1.First Node 2.Last Node 3.Intermediate Nodes
- In Singly Linked List access is given only in one direction thus Accessing Singly Linked is Unidirectional.
- We can have multiple data fields inside Node but we have only single Link for next node.
Node Structure for Singly Linked List :
struct node { int data; struct node *next; }start = NULL;
In the above node structure we have defined two fields in structure –
No | Field | Significance |
---|---|---|
1 | data | It is Integer Part for Storing data inside Linked List Node |
2 | next | It is pointer field which stores the address of another structure (i.e node) |

Explanation of Node Structure :
- We have declared structure of type NODE, i.e we have created a Single Linked List Node.
- A Node in general language is a Structure having two value containers i.e [Square box having two Partitions]
- One value container stores actual data and another stores address of the another structure i.e (Square box having two partitions)
- We have declared a structure and also created 1 very first structure called "Start".
- Very first node "Start" contain 1 field for storing data and another field for address of another structure
- As this is very first node or Structure, we have specified its next field with “NULL” value.